In the world of data science, one of the most valuable skills you can learn is how to scrape data from a website Python. Web scraping allows you to extract data from websites, which you can then use for various applications like research, analysis, or machine learning. In this article, we’ll guide you through the process of how to web scrape with Python by explaining the necessary tools, libraries, and techniques to help you get started.
Web scraping using Python is not only an essential skill for data scientists but also for anyone interested in automating the collection of information from the web. Whether you’re pulling product details from e-commerce sites, news articles from blogs, or financial data from market websites, Python is a go-to tool for web scraping and this Python web scraping tutorial will teach you all the essential things you need to learn.
Let’s dive into the basics of Python web scraping, break down the tools involved, and walk you through building your own web scraper in Python.
How to Scrape Data from a Website Python for Data Science (Brief Overview)
- How to send HTTP requests to websites using Python’s requests library.
- How to parse HTML content with BeautifulSoup for easy data extraction.
- Techniques to extract specific data from websites, such as headlines or articles.
- How to organize scraped data into a structured format like CSV using Pandas.
- How to handle dynamic content with Selenium when data is loaded via JavaScript.
- Best practices for building a robust web scraper Python for automated data collection.
- Legal considerations and ethical guidelines to follow while web scraping.
What is Web Scraping and Why Use Python?
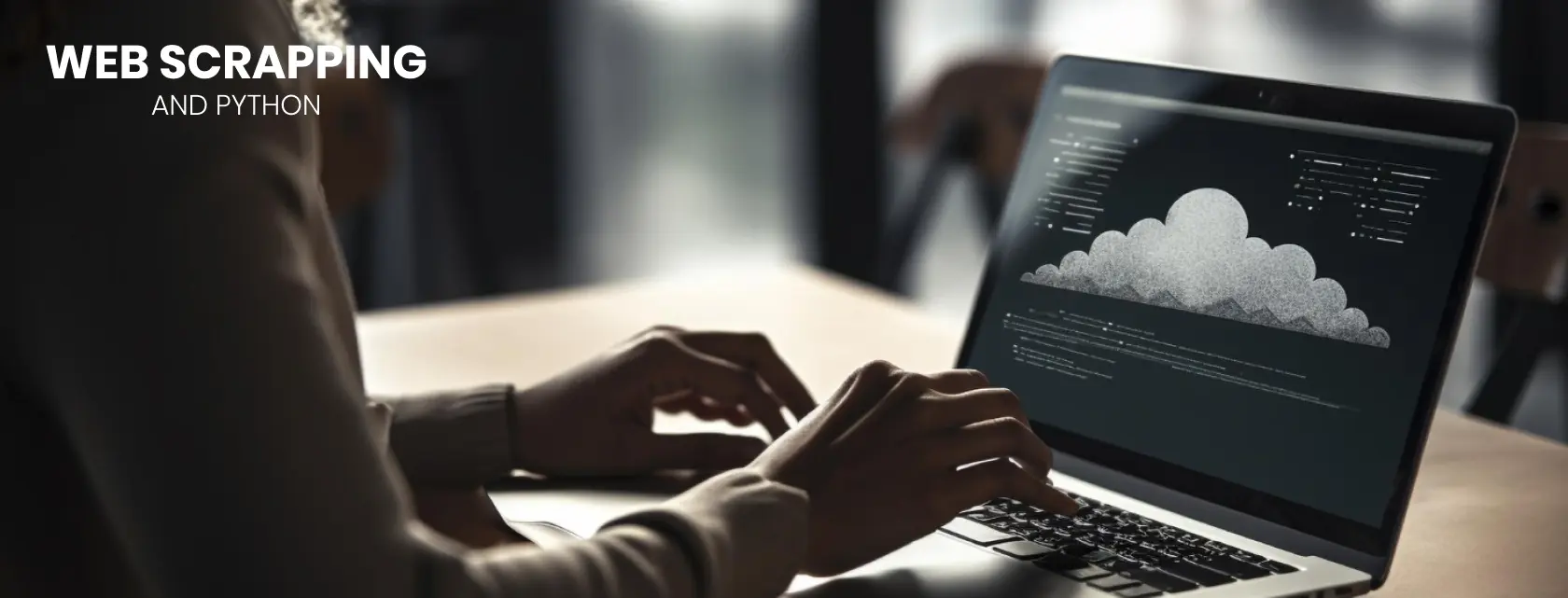
Web scraping is the process of automatically extracting large amounts of data from websites. Python is a perfect choice for this task because of its simplicity, powerful libraries, and active community. Instead of manually copying and pasting data, you can automate the process and extract structured data directly from the web pages.
For data science, scraping web data is often the first step in gathering raw data for analysis or training machine learning models. By using web scraping Python techniques, you can gather data on anything from social media posts to scientific research papers.
Tools You’ll Need for Web Scraping in Python
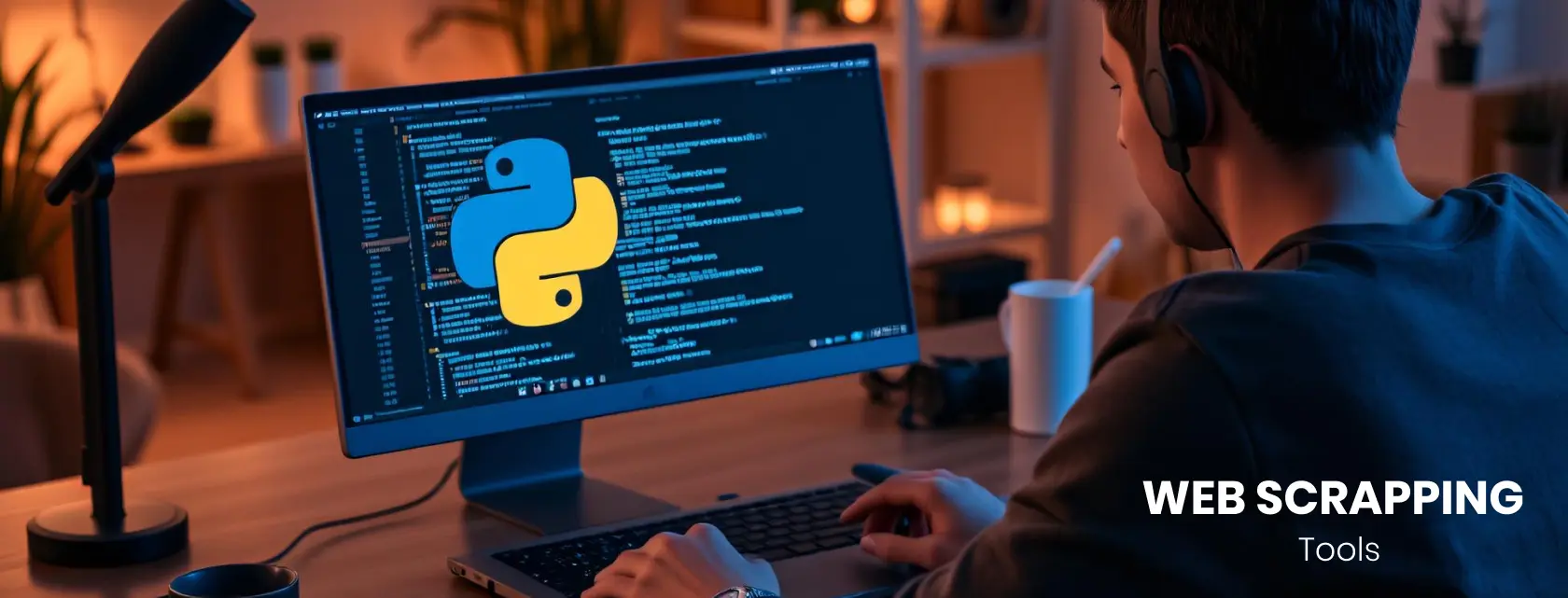
Before you start how to webscrape with Python, you’ll need to install a few libraries. These tools are crucial for navigating, extracting, and processing data from websites.
Requests: This library allows you to send HTTP requests to a webpage and receive the response. It’s perfect for retrieving the HTML content of the webpage.
BeautifulSoup: This Python library helps you parse HTML and XML documents. It makes it easy to navigate the HTML structure and extract specific elements.
Pandas: While not directly related to scraping, pandas is great for organizing and manipulating the data you scrape. You’ll likely want to store the data in a structured format like a CSV or DataFrame.
Selenium (optional): If you need to scrape dynamic content (data loaded via JavaScript), Selenium allows you to interact with web pages like a browser, letting you retrieve dynamically generated data.
How to Scrape a Website Using Python (Step-by-Step)
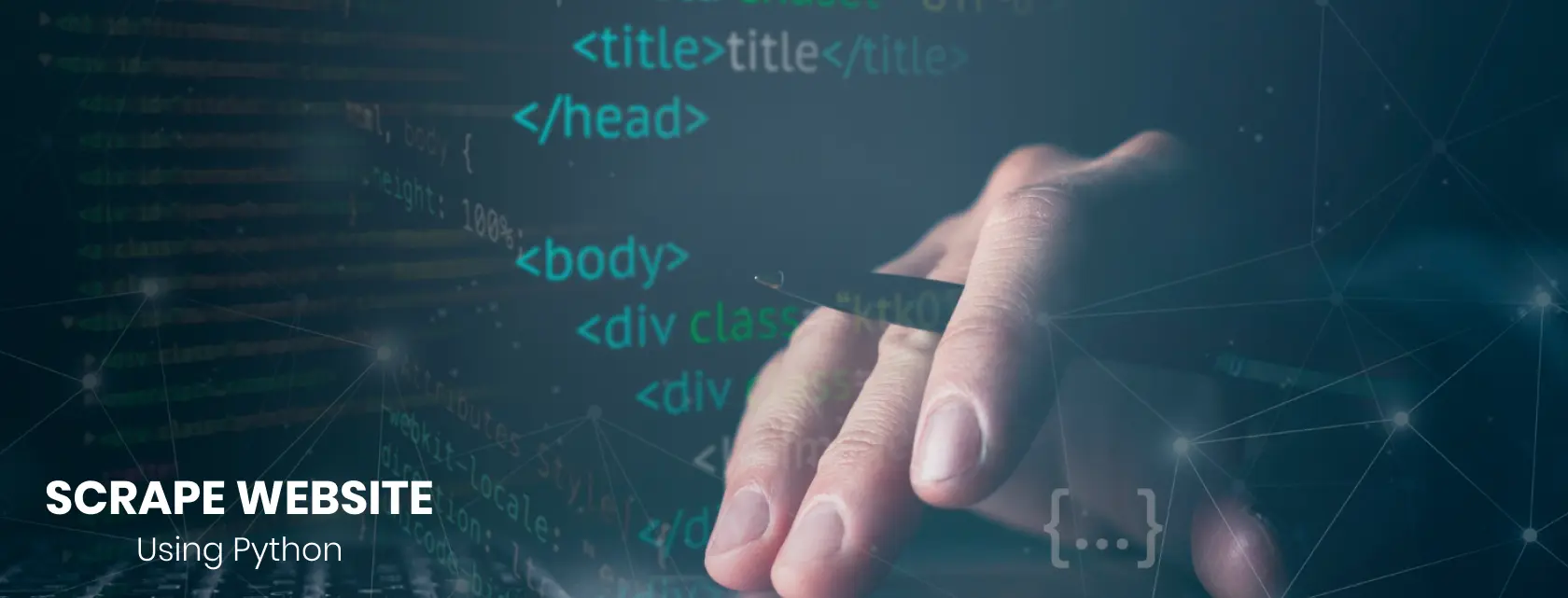
Now that you have the necessary tools installed, let’s walk through the process of creating a basic web scraper Python to collect data from a website. In this example, we’ll scrape a simple HTML page for demonstration purposes.
If you want to learn more about web scraping, enroll in a Python course on FastLearner.ai.
Step 1: Send an HTTP Request to the Website
The first step in any web scraping project is to send an HTTP request to the website you want to scrape. This can be done using the requests library.
import requests
url = 'https://example.com'
response = requests.get(url)
# Check if the request was successful (status code 200)
if response.status_code == 200:
print("Successfully fetched the page")
else:
print("Failed to retrieve the page")
Step 2: Parse the HTML with BeautifulSoup
Once you have the HTML content of the page, you can use BeautifulSoup to parse it. BeautifulSoup helps you search through the HTML structure and extract the elements you’re interested in.
from bs4 import BeautifulSoup
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
# Print the formatted HTML content
print(soup.prettify())
Step 3: Extract the Data
Now that the HTML is parsed, you can easily extract specific elements. For example, let’s say you want to scrape all the headlines from a news website. You can find the headline elements by searching for their HTML tags and classes.
# Find all headline elements (assuming they are in
#tags with a class 'headline')
headlines = soup.find_all('h2', class_='headline')
# Loop through the headlines and print the text content
for headline in headlines:
print(headline.text.strip())
Step 4: Organize the Data
After extracting the data, you’ll probably want to organize it into a structured format like a CSV. Pandas is great for this. Let’s save our scraped data to a CSV file.
import pandas as pd
# Store the headlines in a list
data = {'headlines': [headline.text.strip() for headline in headlines]}
# Convert the list to a pandas DataFrame
df = pd.DataFrame(data)
# Save the data to a CSV file
df.to_csv('headlines.csv', index=False)
Step 5: Handling Dynamic Content (Using Selenium)
If the website you’re scraping uses JavaScript to load content dynamically, you might need to use Selenium to interact with the page and retrieve the data. Here’s how you can set up a basic Selenium web scraper:
Setting Up Selenium for Web Scraping
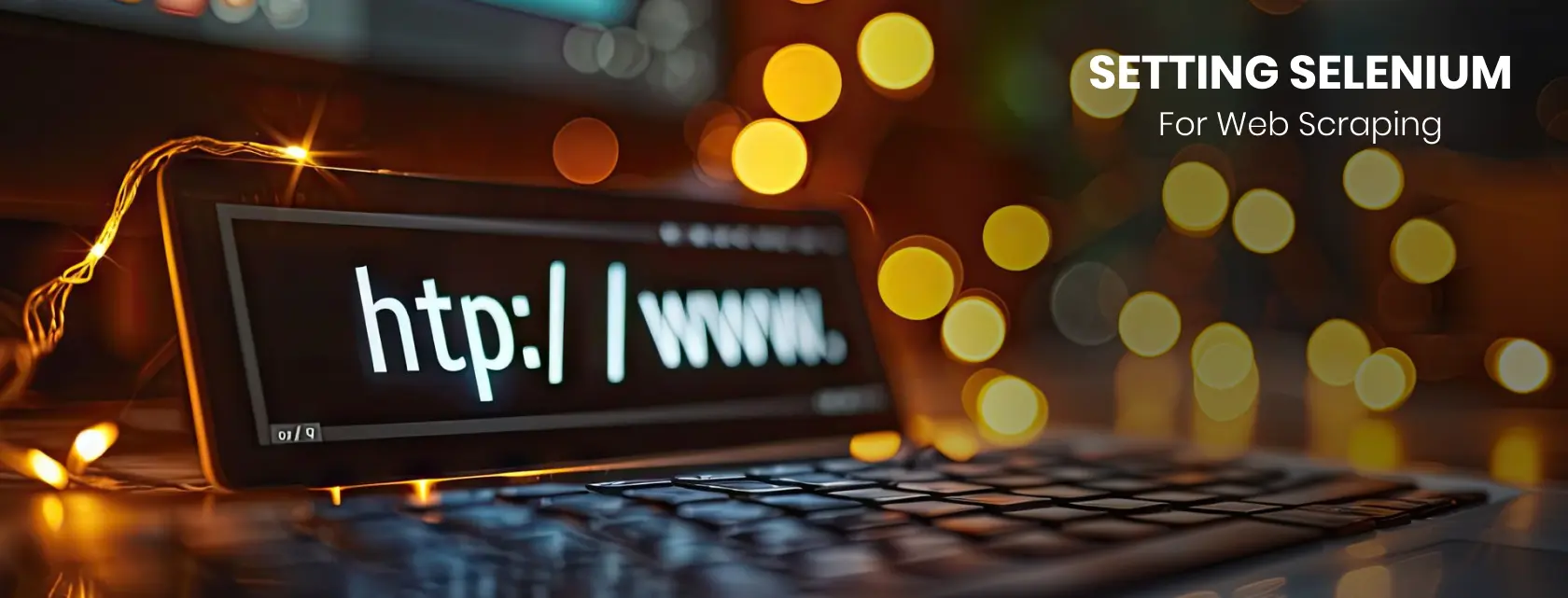
Selenium is a powerful tool for automating web interactions and scraping dynamic content from websites. Follow this step-by-step guide to install Selenium, set up ChromeDriver, and create a web scraper using Python.
Step 1: Install Selenium
If you haven’t already installed Selenium, you can do so using the following command:
pip install selenium
Step 2: Download a WebDriver
Selenium requires a WebDriver to control a browser. The most commonly used WebDriver is for Google Chrome.
To download ChromeDriver that matches your Chrome version, visit:
➡️ ChromeDriver Download Page
Make sure the version of ChromeDriver matches your installed Google Chrome version to avoid compatibility issues.
Step 3: Write a Web Scraper with Selenium
Once Selenium and ChromeDriver are set up, you can write a simple web scraper. Below is an example script that interacts with a web page and retrieves dynamic content using Selenium.
Python Code for Selenium Web Scraper
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
import time
# Set up the WebDriver (this will automatically download the correct ChromeDriver)
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Open the website
url = ‘https://example.com’
driver.get(url)
# Wait for JavaScript content to load (you may need to adjust this based on the site)
time.sleep(5) # Wait for 5 seconds (adjust depending on your website)
# Find the elements you’re looking for, for example, headlines
headlines = driver.find_elements(By.CLASS_NAME, ‘headline’)
# Loop through the headlines and print the text content
for headline in headlines:
print(headline.text.strip())
# Close the browser once you’re done
driver.quit()
Understanding the Code
✔ Initialize WebDriver – The script uses webdriver.Chrome()
to launch a Chrome browser instance.
✔ Open a Website – driver.get(url)
loads the specified webpage.
✔ Wait for Dynamic Content – time.sleep(5)
ensures JavaScript-rendered content loads before scraping.
✔ Extract Data – driver.find_elements(By.CLASS_NAME, 'headline')
finds elements with the class name “headline”.
✔ Loop Through Elements – The script iterates through found elements and prints their text.
✔ Quit Browser – driver.quit()
closes the browser after execution.
Concluding Thoughts - How to Scrape Data from a Website
So, how to scrape data from a website Python for data science? All the answers are discussed in the form of steps in this guide.
We explored the fundamentals of web scraping using Python, a critical skill for data scientists and anyone interested in automating the extraction of information from websites. We covered essential tools such as Requests, BeautifulSoup, Pandas, and Selenium to help you get started with scraping data. Following the step-by-step guide, you learned how to send HTTP requests, parse HTML content, extract relevant data, and organize it into a structured format like CSV.
We also addressed how to handle dynamic content that loads via JavaScript using Selenium, ensuring you can scrape data from modern, interactive websites. Whether you’re scraping static or dynamic content, Python provides the versatility and power needed to gather data efficiently for various purposes, including research, analysis, or machine learning projects.
By following this guide, you now know to start building your own web scraper Python and leverage it for any data science or automation tasks that involve web data. Learning how to scrape a website using Python will enhance your ability to gather valuable insights from the web, saving time and opening up new possibilities for data analysis. Happy scraping!
FAQs About How to Scrape Data from a Website Python for Data Science
How to scrape data from a website using Python?
To scrape data from a website using Python, send an HTTP request to the webpage using the requests library, parse the HTML content with BeautifulSoup, and extract the relevant data. You can also use Selenium for scraping dynamic content that loads via JavaScript.
How to scrape articles from a website in Python?
To scrape articles, use BeautifulSoup to parse the HTML and locate the article content by targeting HTML tags like <article> or specific class names. After extracting the article text, you can organize it in a structured format such as a CSV file.
How do I scrape data from an entire website?
To scrape data from an entire website, you need to send requests to multiple pages (using pagination or URLs) and extract the data from each one. Automate the process with a loop, and use BeautifulSoup to parse and collect the data.
Is web scraping illegal?
Web scraping itself is not inherently illegal, but it can be subject to legal restrictions. It’s important to check a website’s robots.txt file and terms of service to ensure you’re complying with its rules. Additionally, scraping for malicious purposes or without permission can lead to legal issues.