In Python, understanding the type of a variable is fundamental to mastering programming.
Whether you’re debugging, designing new functions, or ensuring your code runs smoothly, knowing how to check data type in Python is essential.
Luckily, Python provides multiple ways to check the data type, from simple built-in functions to more advanced techniques.
So, how to check the type of a variable in Python and master data types like a pro?
This article will guide you through the different methods, ensuring you become a pro at identifying variable types and optimizing your code.
How to Check the Type of a Variable in Python (Brief Overview)
- Learn about common data types like int, float, str, list, and more.
- Explore the type() function for quick and reliable type identification.
- Discover how to verify if a variable belongs to a specific class or subclass.
- Learn how to display variable types during the debugging process.
- Understand how to execute type-specific operations using conditional statements.
- Recognize the importance of type checks for debugging, performance optimization, and error prevention.
Understanding Python Data Types
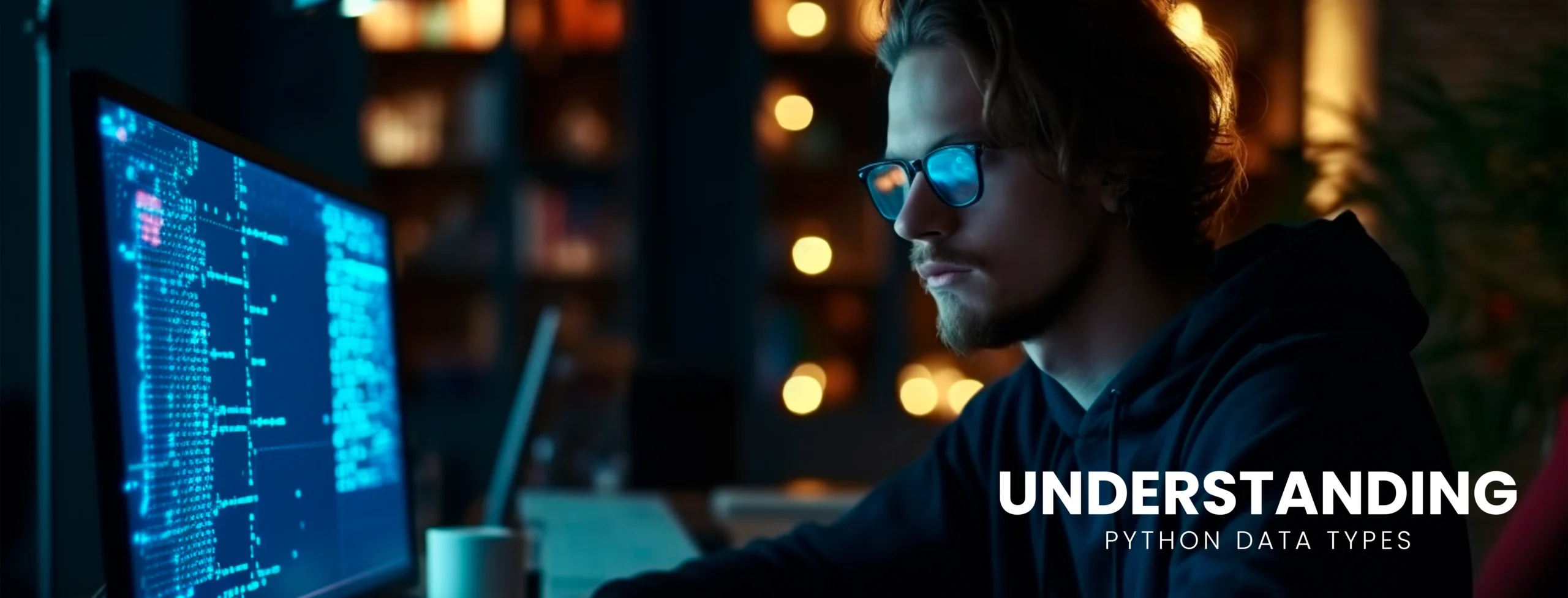
Before diving into how to check the type of a variable in Python, it’s important to understand the concept of data types. Every variable in Python holds a value, and this value belongs to a specific data type. Common data types in Python include:
- int: Integer values, such as 5, -3, or 100
- float: Floating-point numbers, like 3.14, 0.001, or -56.32
- str: Strings, such as “hello” or “Python”
- list: Lists, which are ordered collections, like [1, 2, 3] or [“apple”, “banana”]
- tuple: Tuples, which are immutable ordered collections, like (1, 2, 3) or (“a”, “b”)
- dict: Dictionaries, which store key-value pairs, like {“name”: “John”, “age”: 30}
- bool: Boolean values, representing True or False
- set: Sets, which are unordered collections with unique elements, like {1, 2, 3} or {“apple”, “banana”}
Each of these data types behaves differently and determines how operations like addition, comparison, or iteration will work. Thus, knowing how to check the type of a variable in Python can save you time by ensuring that your code behaves as expected.
If you want to learn more about this programming language, read our article “What Can You Do with Python? From Web Development to AI, Explore Its Limitless Potential” in the FastLearner blog.
Built-In type() Function: The Most Common Way to Check Variable Type in Python
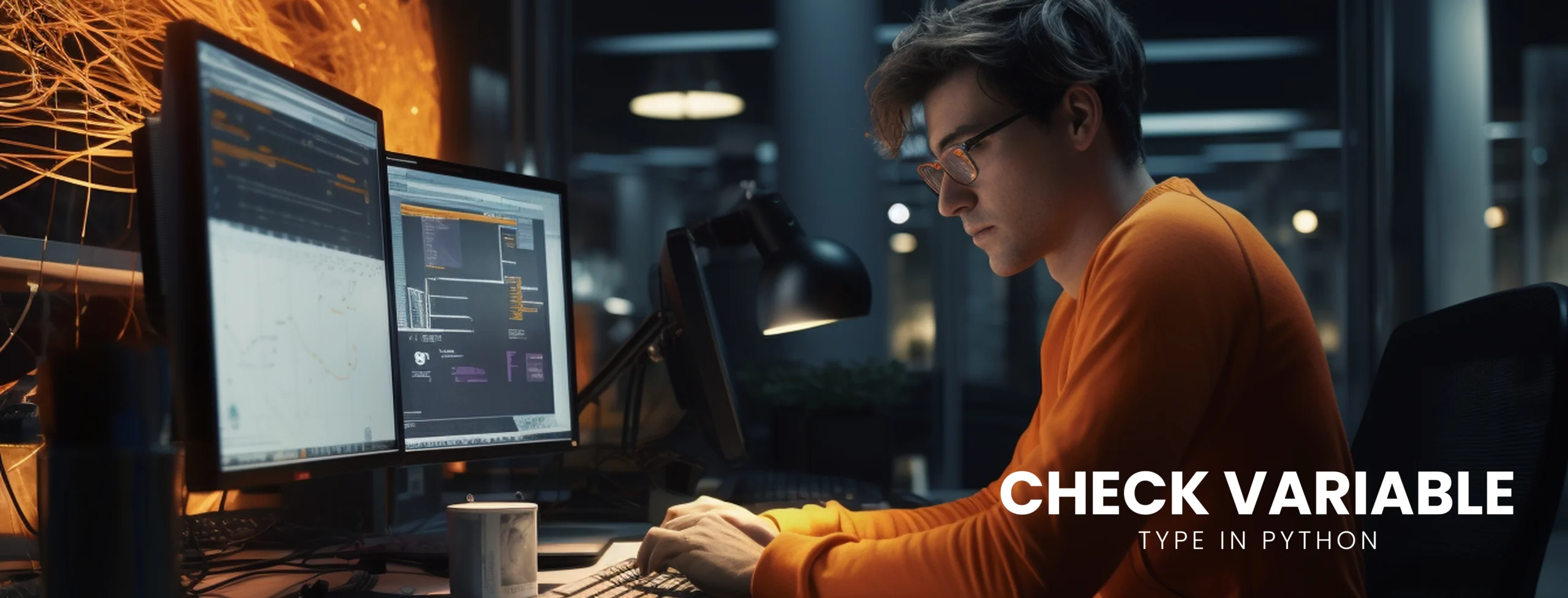
The simplest and most commonly used method to check the type of a variable in Python is by using the built-in type() function. This function Python return type of the object passed to it.
When you use type(), Python will evaluate the variable and give you the class to which that object belongs. For example, if you pass an integer to type(), it will return int, which is the type class for integers in Python. This function is useful because it works with all Python data types, making it a reliable tool for identifying variable types quickly.
The type() function is versatile and can be used in various scenarios. Whether you’re checking a variable’s type for debugging purposes or preparing for more advanced operations, it serves as a quick and efficient way to get the job done. It’s important to note that type() is not limited to built-in data types; you can also use it to check the types of custom classes and objects you define in your code.
The isinstance() Function: A More Specific Type Check
While type() is useful, sometimes you need to check whether a variable is an instance of a particular class or a subclass. In such cases, the isinstance() function is more appropriate.
Unlike type(), which only checks if an object is exactly of a specific type, isinstance() allows you to check whether an object is an instance of a class or a subclass of that class. This is particularly useful in object-oriented programming when dealing with class hierarchies.
For example, when working with user-defined classes, isinstance() can help you verify if a variable is an instance of a class or its subclass, even if the actual object is of a different class but shares a common base class. This feature provides greater flexibility and is widely used in Python for checking inheritance and class relationships.
Python print Variable Type
Another way to check the type of a variable is by using the print() function in combination with the type() function. While this method isn’t as formal as directly using type() for type checks, it can be handy for quickly displaying a variable’s type, especially during the debugging process.
The print() function simply outputs the value of its argument to the console. When combined with type(), it allows you to display the variable’s type along with any other relevant information. This method is particularly useful when you want to quickly inspect a variable’s type without setting up formal checks in your code.
If you want to learn more about Python and programming to acquire quick learning skills, visit Fast Learner courses.
Checking the Type of a Variable Using type() in Conditional Statements
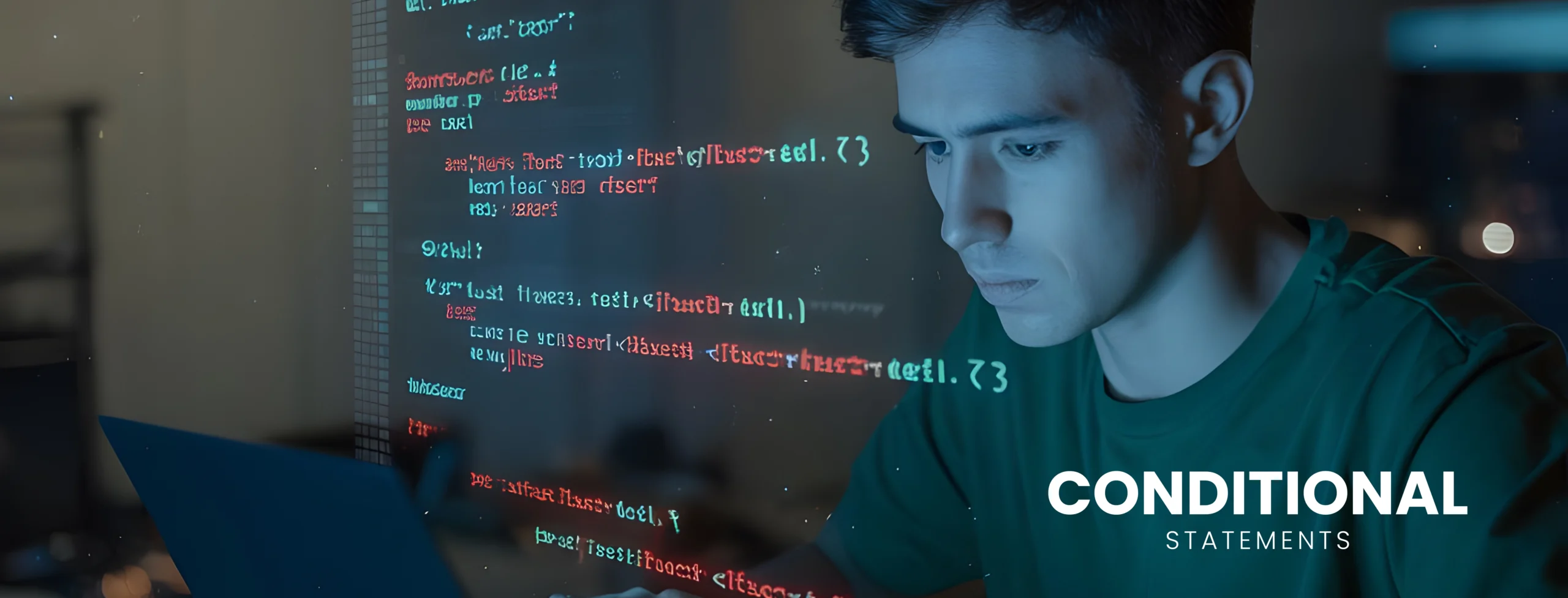
In many situations, you may need to perform actions based on a variable’s type. The type() function can be used inside conditional statements to trigger specific logic depending on the type of a variable.
This approach allows your program to handle different data types in unique ways. For example, if you’re writing a function that works with multiple types of input, you may want to execute different code for integers, strings, or lists. By checking the variable type, you can ensure that your program handles each case appropriately.
Using type() in this way can help make your code more flexible and robust, as it enables the program to respond to the type of input it’s given.
The id() Function: Get the Object’s Identity
While not directly related to checking types, the id() function provides additional useful information about a variable in Python. The id() function returns a unique identifier for an object, which can be helpful when debugging complex scenarios involving variable references and object identities.
The value returned by id() represents the object’s location in memory. This function can be particularly useful when trying to track how variables are referenced and to confirm whether multiple variables point to the same object. However, id() should not be used for determining the type of an object; instead, it complements type() by providing insight into an object’s identity.
Why It’s Important to Check Variable Types
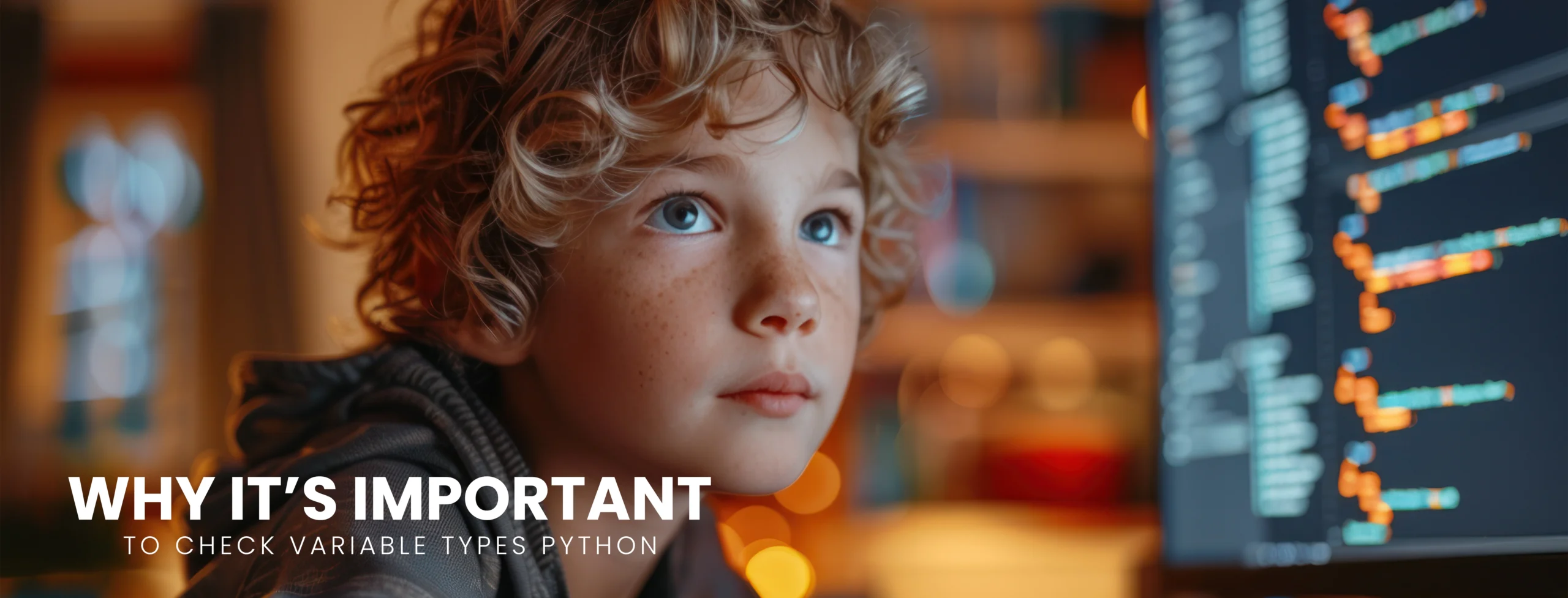
Knowing how to check the type of a variable in Python is crucial for several reasons:
- Debugging: When you’re troubleshooting errors, checking types helps identify issues that arise due to incompatible operations. For example, trying to add a string to an integer will cause an error if the types are not handled properly.
- Code Optimization: By understanding the data type, you can optimize algorithms for better performance. For instance, processing integers is typically faster than processing strings.
Type-Specific Operations: Some operations, such as mathematical computations or string manipulation, only work with specific data types. Ensuring the type is correct prevents runtime errors and logical bugs.
Conclusion - How to check the type of a variable in Python: Master data types like a pro?
In this article, we’ve explored how to check the type of a variable in Python using various methods, such as the type() function, isinstance(), and using type() in conditional statements. These methods are invaluable for debugging, optimizing, and ensuring the correctness of your Python code.
So, how to check the type of a variable in Python and master data types like a pro?
By incorporating these tools and techniques, you’ll become more adept at handling variables, and your Python code will run more smoothly and predictably.
Whether you’re just starting or you’re a seasoned developer, knowing how to check the type of a variable in Python is a vital skill in your programming toolkit.
With the ability to check and verify types easily, you can confidently write efficient, error-free Python code that performs exactly as expected.
Frequently Asked Questions
1. How do you check the type of a variable in Python?
Use the type() function to quickly check the type of any variable. For example, type(variable) returns the class of the object stored in the variable.
2. How to check if a variable is of type list in Python?
You can use the isinstance() function to check if a variable is a list. For example, isinstance(variable, list) returns True if the variable is a list, and False otherwise.
3. What is type() in Python?
The type() function is a built-in method in Python that returns the type or class of an object. It’s widely used for identifying variable types during debugging or conditional logic.
4. How do you check the data type of a variable?
You can check the data type of a variable in Python using the type()
function.